Decomposition
Decomposition
Decomposition is the process of breaking a problem down into its component parts.
For instance if you are designing a hangman style game then your program might be broken down into:
- Displaying the start menu
- Asking for a letter to be entered
- Displaying the gallows
- Displaying a game over screen.
Each of these components can be broken down further into sub components,
- Displaying the start menu:
- Print out all the menu options
- Ask the user for an input
- Redirect the user to the correct screen depending on their choice.
In programming we often use functions, procedures and modules to decompose the problem down into separate sub problems. We can take a structured approach to decomposition, for instance by using stepwise refinement.
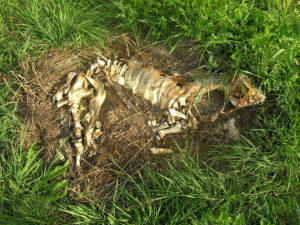
When animal decomposes, its body breaks down into separate component parts. Source: HBreton19
Pattern Recognition
Pattern Recognition
A key skill in becoming a programmer is being able to pick out the patterns present in both problems to be solved and the data the problems work on. Recognition of patterns allows algorithms to be adapted so that multiple problems can be solved more efficiently.
Example 1
Example 1
player1name = input("What is player 1's name?") player2name = input("What is player 2's name?") player3name = input("What is player 3's name?") player4name = input("What is player 4's name?") player5name = input("What is player 5's name?")
In the example above there is a clear pattern and a solution can easily be programmed:
names = [] for n in range(5): names.append(input("What is player",n+1,"'s name?"))
While the above example only saves a couple of lines of code, if the number of players is increased you need only change the number 5.
Example 2
Example 2
while True: height = input("How tall are you in cm?") if ans.isdigit(): if 50 <= int(height) <= 300: print("please input a valid number between 50 and 300") continue break else: print("please input a valid number between 50 and 300") while True: age = input("How oldare you in years?") if ans.isdigit(): if not (0 <= int(height) <= 110): print("please input a valid number between 0 and 110") continue break else: print("please input a valid number between 50 and 300")
This example is a little more complex and here functions can be useful in dealing with patterns.
def get_integer(minval,maxval,question): while True: ans = input(question) if ans.isdigit(): if minval <= int(ans) <= maxval: break print("please input a number between" , minval , "and" , maxval) age = get_integer(0,110,"How old are you?") height = get_integer(50,300,"How tall are you?")
This improved version has a number of benefits:
- The less code is required to solve the problem
- The code of more readable because the code has been split into functions.
- The code is more reusable because it has been generalized to solve a number of similar problems.
Abstraction
Abstraction
Abstraction is the process of simplifying a problem and throwing unnecessary detail until we are left with only the information that we need to solve a problem.
What information is deemed necessary or otherwise depends wholly at the problem at hand. For example if we are storing data about pupils in a school database then we might need to store their education history, whereas in a database for a hospital this data would not be needed.
The abstraction of problems involves making choices such as what data type to use (e.g. integer versus real) or whether to store data in an array or a dictionary.
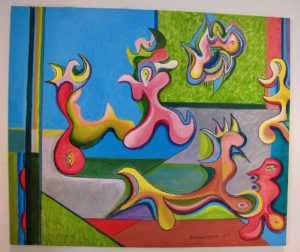
In abstract art the artist only seeks to record the details that are important to them, discarding unnecessary detail. Source: 20thCenturyArtEnthusiast